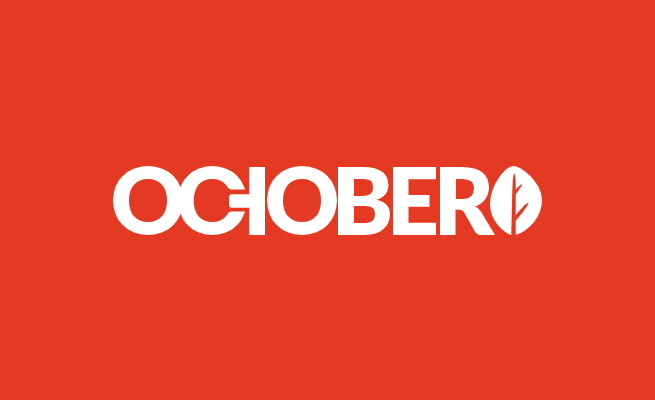
Components in October CMS can be used for custom output, such as JavaScript snippets, dynamic content from a web service or models. Components can also handle AJAX requests or other incoming data. In this tutorial, I will simply show you how to register a new component and output a list with records from the users table.
First of all, you have to register a new component in your Plugin.php file. For example, users.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
<?php namespace Playground\Sandbox; use System\Classes\PluginBase; use System\Classes\SettingsManager; class Plugin extends PluginBase { /** * Register Component * * @return array */ public function registerComponents() { return [ 'Playground\Sandbox\Components\Users' => 'users' ]; } } |
Create the following structure within the components folder which must contain the file Users.php and users/default.htm.
1 2 3 4 5 6 7 8 |
. └── playground └── sandbox ├── Plugin.php ├── components │ ├── Users.php │ └── users │ └── default.htm |
Below you will find the content for the default.htm file which will basically output a list of all users.
1 2 3 4 5 |
<div class="list-users"> {% for person in __SELF__.getPeople %} {{ person.username }} {{ person.email }} {% endfor %} </div> |
The Users.php file only requires a method getPeople and a reference to the new model, called UsersModel.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<?php namespace Playground\Sandbox\Components; use Cms\Classes\ComponentBase; use Playground\Sandbox\Models\Users as UsersModel; class Users extends ComponentBase { /** * Define component details. * * @return array */ public function componentDetails() { return [ 'name' => 'playground.sandbox::lang.plugin.name', 'description' => 'playground.sandbox::lang.plugin.description' ]; } public function getPeople() { return (new UsersModel())->getPeople(); } } |
In order to access a model, you must create one within the models folder.
1 2 |
├── models │ └── Users.php |
For test purposes, I simply return all records from the users table.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<?php namespace Playground\Sandbox\Models; use Model; /** * Class Users * @package Playground\Sandbox\Models */ class Users extends Model { /** * @var string The database table used by the model. */ public $table = 'users'; /** * Return all records from users table. * * @return mixed */ public function getPeople() { return self::all(); } } |
Now you can use the new component anywhere in your template files. I recommend to use [users] in your parent template file, because it will allow you to use the component in your child templates.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
description = "Default layout" [users] == <!doctype html> <html lang="en"> <head> </head> <body> <section id="body"> <div class="wrapper"> {% component 'users' %} </div> </section> {% scripts %} {% framework extras %} </body> </html> |
After a page reload, should you immediately see the list.
For more information, check out https://octobercms.com/docs/cms/components. The source code of this tutorial can be found on https://github.com/tobias-forkel/october-cms-playground