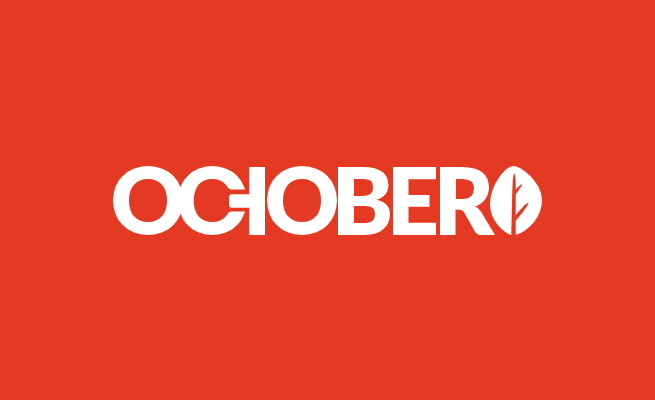
At some point you probably want to make your custom plugin for October CMS configurable and allow backend users to make changes within the plugin space. October CMS has a simple and intuitive backend navigation, which you can easily extend in no-time. Let’s assume you have a custom plugin that calls an external API. Here is how you can store API credentials by using the Settings Manager.
First of all, you must register the settings in your Plugin.php file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
<?php namespace Playground\Sandbox; use System\Classes\PluginBase; use System\Classes\SettingsManager; class Plugin extends PluginBase { /** * Register Settings * * @return array */ public function registerSettings() { return [ 'settings' => [ 'label' => 'playground.sandbox::lang.settings.label', 'description' => 'playground.sandbox::lang.settings.description', 'icon' => 'icon-flask', 'category' => SettingsManager::CATEGORY_SYSTEM, 'class' => 'Playground\Sandbox\Models\Settings', 'keywords' => 'playground sandbox other keyswords', ] ]; } } |
After that you must create a Settings.php file with the following content inside your models folder.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
<?php namespace Playground\Sandbox\Models; use Model; /** * Class Settings * @package Playground\Sandbox\Models */ class Settings extends Model { /** * @var array */ public $implement = ['System.Behaviors.SettingsModel']; /** * @var string */ public $settingsCode = 'playground_sandbox_settings'; /** * @var string */ public $settingsFields = 'fields.yaml'; // Set defaults public function initSettingsData() { $this->enabled = 0; $this->api_key = ''; } } |
If you define default values inside the method initSettingsData, make sure the above fields match with the definitions inside the fields.yaml file.
1 2 3 4 5 6 7 8 9 10 11 12 |
tabs: fields: enabled: label: playground.sandbox::lang.settings.enabled comment: playground.sandbox::lang.settings.enabled_comment tab: playground.sandbox::lang.settings.tab_default type: checkbox api_key: label: playground.sandbox::lang.settings.api_key comment: playground.sandbox::lang.settings.api_key_comment tab: playground.sandbox::lang.settings.tab_default type: text |
Once the fields.yaml file is created, you should see a new form within the new tab.
Now you can access the settings values within your controller, console or component by implementing the following model.
1 |
use Playground\Sandbox\Models\Settings; |
For test purposes, I created a new controller and implemented the above model to get access to the API key.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
<?php namespace Playground\Sandbox\Controllers; use Illuminate\Routing\Controller; use Playground\Sandbox\Models\Settings; class Swing extends Controller { /** * @var Plugin settings */ protected $settings; /** * Sandbox constructor. */ public function __construct() { $this->settings = Settings::instance(); } /** * @param $params */ public function index($params) { try { $enabled = $this->settings->enabled; if ($enabled) { // Use settings values var_dump($this->settings->api_key); } } catch (\Exception $ex) { $ex->getMessage(); } } } |
Here you go.
The entire source code can be found on https://github.com/tobias-forkel/october-cms-playground.